I got my hands on a private beta of Pagoda Box. It is a platform that you can deploy your PHP applications to. I gave it a brief look around and I have to say it’s pretty sweet.
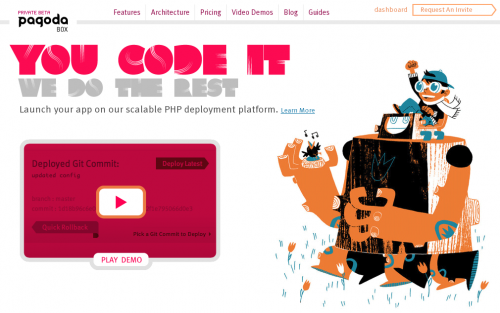
Right after you register and get access to your dashboard, you can add applications. Applications are cloned from GitHub repositories. Both public and private repositories are supported. Once you add an application, you can access it at http://your-app-name.pagodabox.com. If you’d rather have your own domain – you can assign it to your application from the dashboard and all that will remain to be done is adding an A-record in your DNS zone. Super easy!
There is more to it, even at this beta stage. Pagoda Box supports a number of PHP frameworks, including all major ones – CakePHP, CodeIgniter, Lithium, Symfony, Zend, and more. You can also optionally have a MySQL database for your application. They even help you out with outgoing email.
On top of that, you have control as to how many instances of the application you want (the more you have, the more requests you can serve at the same time, and the more you’ll have to pay). There are statistics of your application performance, requests, and a few other parameters (I’m sure those will grow together with the project).
I’ll admit, I am too used to hosting my projects on my own servers to take immediate advantage of Pagoda Box. But I am now seriously considering which projects I can move out of my server and into this platform. It just makes things so much easier. Deploying and re-deploying works wonders for any GitHub commit of your project. Initial resources that one usually needs to try an idea out are free of charge. If the idea picks up, the prices are more than reasonable (and comparable to other hosting solutions).
Out of those things that I consider necessary, I haven’t see any mentioning of files (uploaded via application, for example), support for build systems (such as Phing), and some sort of common library of frequently used code (PEAR modules, for example). But I’m sure that either I simply didn’t look for these hard enough, or they will be added in the future.
If you are a PHP developer or involved with PHP source on GitHub in any other way, I suggest you try it out. You can request a private beta invite directly from Pagoda Box website. Or, if you prefer, I can send you one (I have about 10 of them left for now). Also watch the demonstration screencasts, and read through other platform features.